Comparing nested Python dictionaries with no hassle
Today I was in need of comparing two Python dictionaries with each other — including nested dictionaries. I searched the web for solutions on how to do that properly, and found a lot of recursive approaches, all trying to respect the various data types Python provides (especially lists and dictionaries, optionally (deeply) nested).
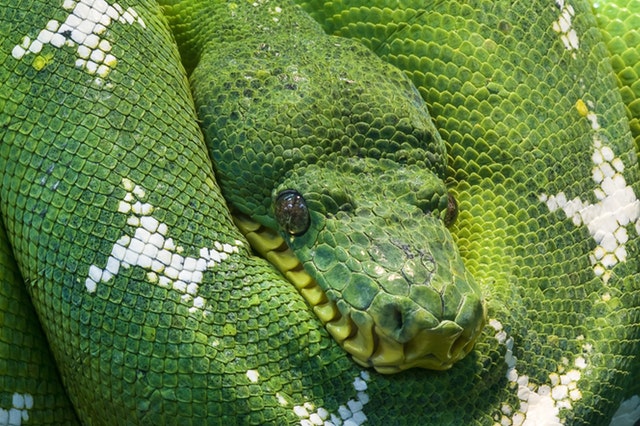
If you are not concerned too much about super lightning-fast speed (read: time-critical environments), you can simply serialize the dictionaries to JSON and compare them. (If in doubt, you can always profile your code.)
The only hurdle is that the dictionary keys need to be sorted, otherwise you get wrong results. Python does not guarantee the order of dictionary keys when iterating them, therefore we have to make sure they are sorted.
This is how it works:
import json dict_ = dict(b=1, a=2, z=3, f=4, e=dict(F=1, C=2)) dump = json.dumps(dict_, sort_keys=True, indent=2) print(dump)
The output is:
{ "a": 2, "b": 1, "e": { "C": 2, "F": 1 }, "f": 4, "z": 3 }
There we go, sorted, and ready to be compared — just drop the indent=2 which I solely used for demonstration purposes.
This article was published on
Dec. 14th, 2016 by
stschindler and is tagged with